- Write a C program to find roots of a quadratic equation. A quadratic equation is a second order equation having a single variable.Any quadratic equation can be represented as ax 2 + bx + c = 0, where a, b and c are constants( a can’t be 0) and x is unknown variable.
- Program to find the roots of Quadratic Equation. Here we will discuss how to find the roots of quadratic equation where it is given that real roots exists for the given equation, using C programming language. Quadratic Equation is equation of the form ax 2 + bx + c = 0, where a, b and c are real numbers and a!= 0. The term b 2-4ac is known.
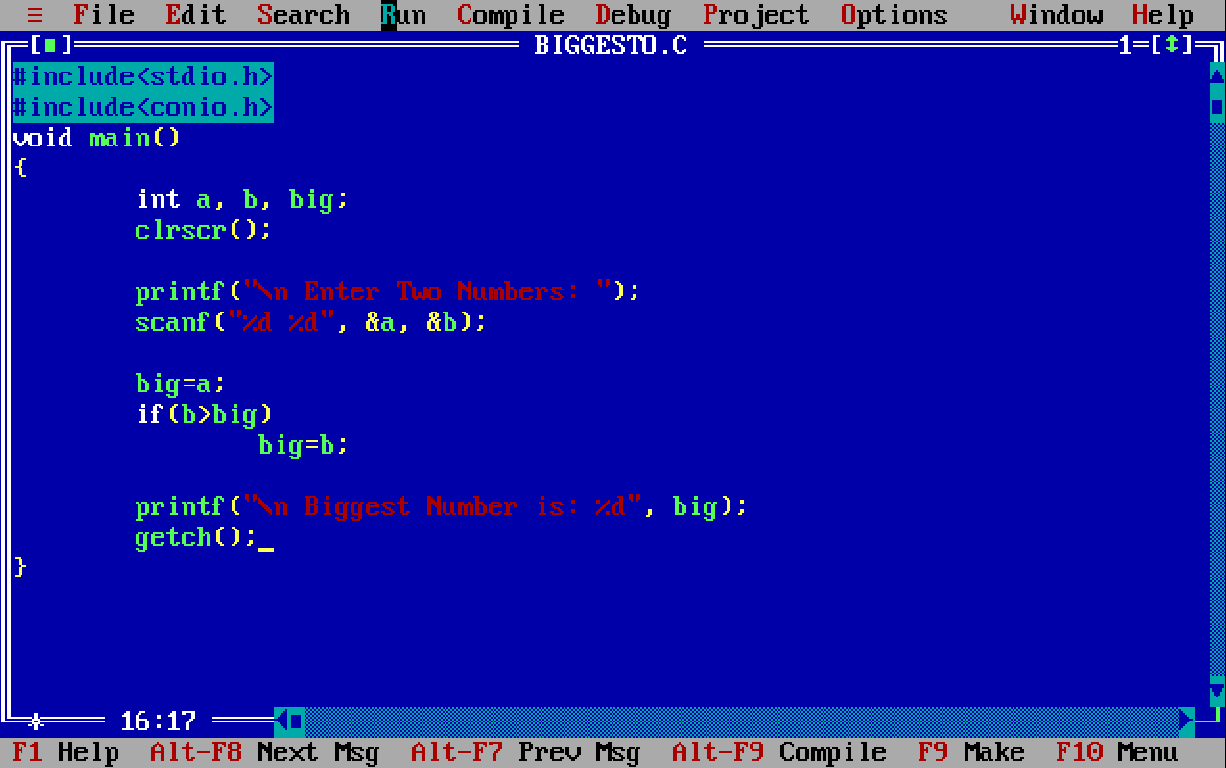
We can further enhance the program to prompt the input values of a, b, c to the user. Right now we have to modify the code for the values. Generate the equation string based on the values of a, b, and c and display the equation string in the output. Take into consideration the imaginary roots if discriminant is less than 0.
C program to find roots of a quadratic equation: Coefficients are assumed to be integers, but roots may or may not be real.
For a quadratic equation ax2+ bx + c = 0 (a≠0), discriminant (b2-4ac) decides the nature of roots. If it's less than zero, the roots are imaginary, or if it's greater than zero, roots are real. If it's zero, the roots are equal.
For a quadratic equation sum of its roots = -b/a and product of its roots = c/a. Let's write the program now.
Roots of a Quadratic equation in C
#include <stdio.h>#include <math.h>
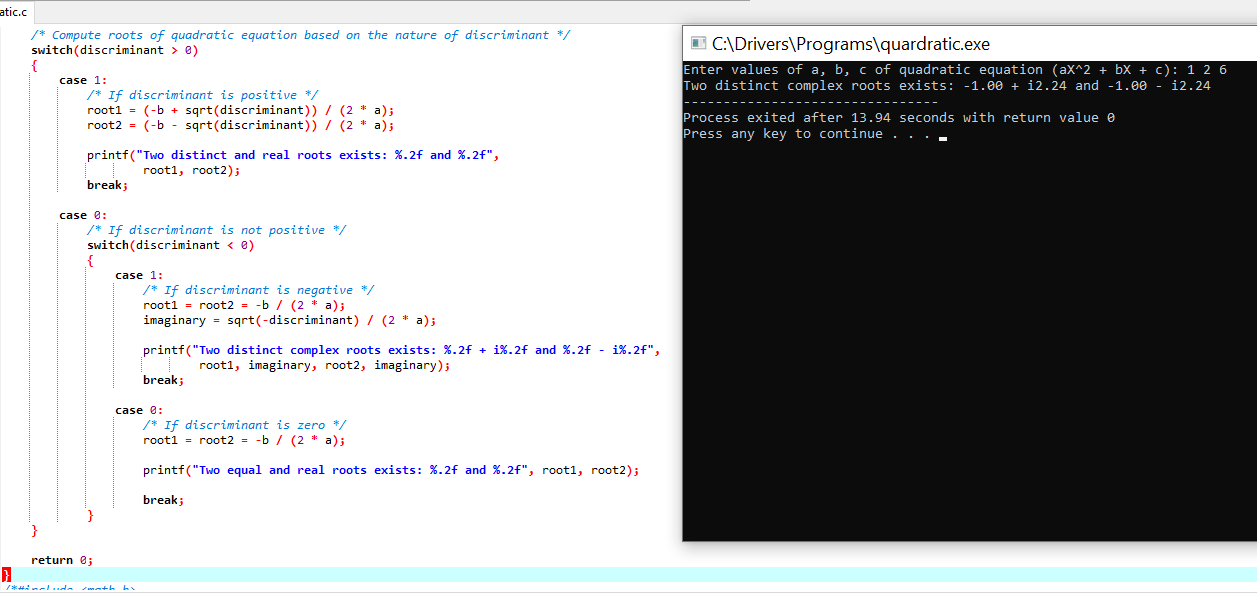
int main()
{
int a, b, c, d;
double root1, root2;
printf('Enter a, b and c where a*x*x + b*x + c = 0n');
scanf('%d%d%d',&a,&b,&c);
d = b*b -4*a*c;
if(d <0){// complex roots, i is for iota (√-1, square root of -1)
printf('First root = %.2lf + i%.2lfn',-b/(double)(2*a),sqrt(-d)/(2*a));
printf('Second root = %.2lf - i%.2lfn',-b/(double)(2*a),sqrt(-d)/(2*a));
}
else{// real roots
root1 =(-b +sqrt(d))/(2*a);
root2 =(-b -sqrt(d))/(2*a);
printf('First root = %.2lfn', root1);
printf('Second root = %.2lfn', root2);
}
return0;
}
- Related Questions & Answers
- Selected Reading
C Program To Find Roots Of Quadratic Equation Using Functions
A quadratic equation is in the form ax2 + bx + c. The roots of the quadratic equation are given by the following formula −
There are three cases −
b2 < 4*a*c - The roots are not real i.e. they are complex
b2 = 4*a*c - The roots are real and both roots are the same.
b2 > 4*a*c - The roots are real and both roots are different
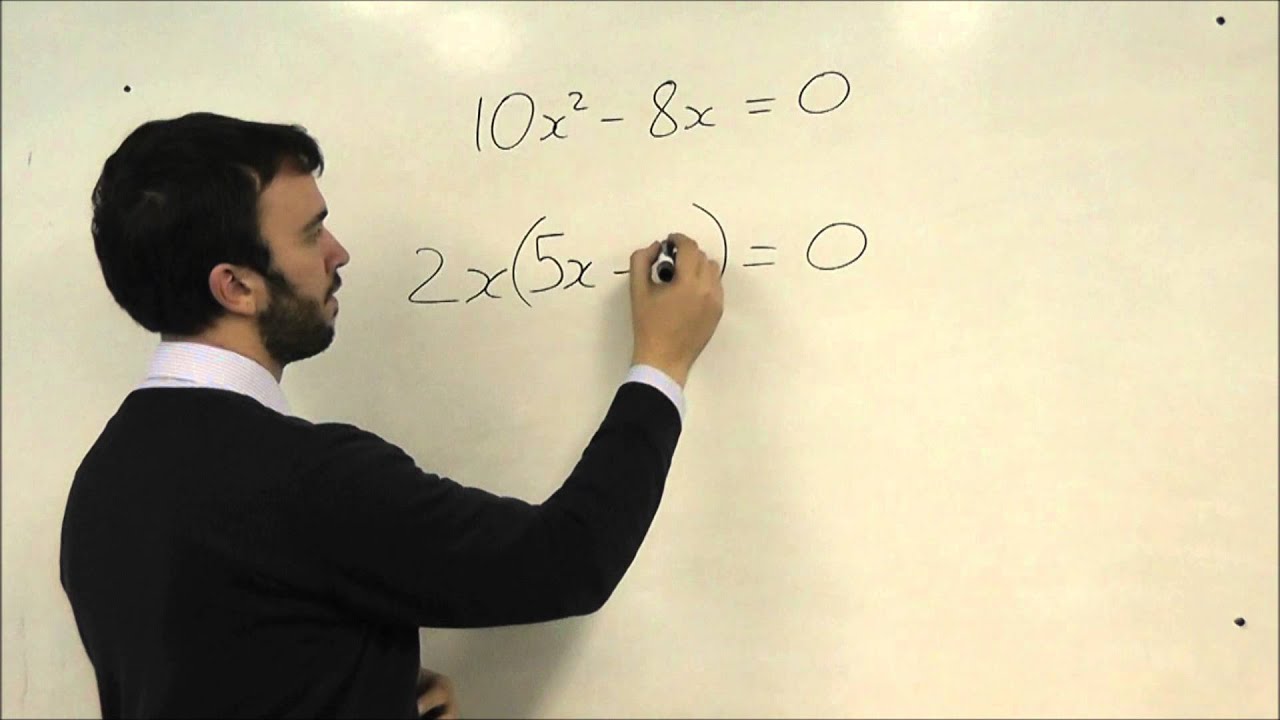
The program to find the roots of a quadratic equation is given as follows.
Example
Output
C Program To Solve Quadratic Equation
In the above program, first the discriminant is calculated. If it is greater than 0, then both the roots are real and different.
This is demonstrated by the following code snippet.
C Program To Get Quadratic Equation
If the discriminant is equal to 0, then both the roots are real and same. This is demonstrated by the following code snippet.
C Program To Find Quadratic Equation
If the discriminant is less than 0, then both the roots are complex and different. This is demonstrated by the following code snippet.